자바 개발자를 위한 코틀린 입문 에 강의 내용을 정리한 내용이다.
코틀린에서 상속을 다루는 방법에 대해서 설명한다.
추상 클래스
Animal.kt1 2 3 4 5 6 7 8
| abstract class Animal( protected val species: String, protected open val legCount: Int, ) {
abstract fun move()
}
|
상위 클래스의 생성자를 바로 호출
1 2 3 4 5 6 7 8 9
| class Cat( species: String ) : Animal(species, 4) {
override fun move() { println(" 고양이가 사뿐 사뿐 걸어가~") }
}
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| class Penguin( species: String ) : Animal(species, 2) {
private val wingCount: Int = 2
override fun move() { println("펭귄이 움직인다~") }
override val legCount: Int get() = super.legCount + this.wingCount
}
|
인터페이스
Flyable.kt1 2 3 4 5 6 7
| interface Flyable {
fun act() { println("파닥 파닥") }
}
|
Swimable.kt1 2 3 4 5 6 7
| interface Swimable {
fun act() { println("어푸 어푸") }
}
|
Penguin.kt1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| class Penguin ( species: String ) : Animal(species, 2), Swimable, Flyable {
private val wingCount: Int = 2
override fun move() { println("펭귄이 움직인다~") }
override val legCount: Int get() = super.legCount + this.wingCount
override fun act() { super<Swimable>.act() super<Flyable>.act() }
}
|
상속 관련 키워드
- final: override를 할 수 없게 한다. default로 보이지 않게 존재
- open: override를 열어 준다.
- abstract: 반드시 override 해야 한다.
- override: 상위 타입을 오버라이드 하고 있다.
소스코드
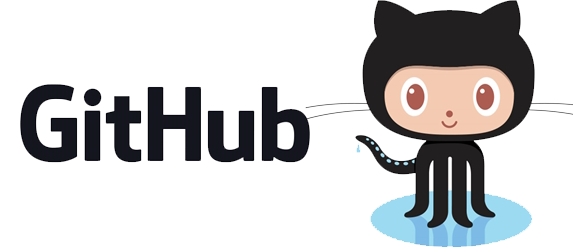
참조